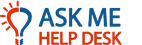 |
|
|
 |
New Member
|
|
May 8, 2009, 03:43 AM
|
|
Add a checkbox item to a listbox at chosen position
How can I add the text from a checkbox into a listbox before some text already in there and after some other text?
As the picture attached shows, I need to add the bakery items after "Turn on, heat" and before the other 3 texts.
Any help with the times would be good as well.
Thanks
|
|
 |
Ultra Member
|
|
May 8, 2009, 05:33 AM
|
|
You can use the Insert method of listbox.Items
Dim InsertStr as string = "This item to be added"
listboxname.Items.Insert(Index, InsertStr)
Remember that Index starts at 0 so if you wish to insert it at the head of the list, Index will be 0.
listbox.Items also has Remove and RemoveAt methods. RemoveAt takes the index of the position. Remove takes the actual object to be removed.
|
|
 |
New Member
|
|
May 8, 2009, 06:40 AM
|
|
Thank you, works well.
Any ideas how to get the times working? The code I have for that section is:
Private Sub btnAdd_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles btnAdd.Click
'adds ticked items to list boxes
Dim chkBakeItem As CheckBox
Dim dttKnot As Integer = 15
Dim intSupreme As Integer = 15
Dim intHawian As Integer = 15
Dim intTank As Integer = 15
Dim intAnzac As Integer = 15
Dim intRocky As Integer = 15
Dim intChocMac As Integer = 15
Dim intDoubleChoc As Integer = 15
Dim intDinner As Integer = 18
Dim intRound As Integer = 18
Dim intLong As Integer = 18
Dim intScotch As Integer = 20
Dim intCheese As Integer = 20
Dim intCheeseAndBacon As Integer = 20
Dim intHamburger As Integer = 20
Dim intFrench As Integer = 20
Dim intVienna As Integer = 22
Dim intSquareWhite As Integer = 25
Dim intSquareWhole As Integer = 28
Dim strInsert As String = gbxBakeryItems.Text
lbxOven1.SelectedIndex.Equals(-2)
If tbxStart.Text = "" Or tbxFinish.Text = "" Then
MessageBox.Show("Please enter Start and Finish times of shift before adding items")
Else : For Each chkBakeItem In gbxBakeryItems.Controls
If chkBakeItem.Checked = True And rdbOven1.Checked = True Then
lbxOven1.Items.Insert(1, (tbxStart.Text + (" ") & (chkBakeItem.Text)))
ElseIf chkBakeItem.Checked = True And rdbOven2.Checked = True Then
lbxOven2.Items.Insert(1, (tbxStart.Text + (" ") & (chkBakeItem.Text)))
ElseIf chkBakeItem.Checked = True And rdbOven3.Checked = True Then
lbxoven3.Items.Insert(1, (tbxStart.Text + (" ") & (chkBakeItem.Text)))
End If
Next
End If
End Sub
End Class
|
|
 |
Ultra Member
|
|
May 8, 2009, 09:06 AM
|
|
So far, you're just entering items into the listbox, and labeling them with the starting time.
Is gbxBakeryItems a groupbox? The line
Dim strInsert As String = gbxBakeryItems.Text
will always be "Bakery items and baking times", and I don't think that's what you want.
Here is a very general idea of how to add items with after a start time.
Dim EventTime As DateTime = DateTime.Parse(tbxStart.Text) ' interprets it as today and the time in "hh:mm"
Dim ElapseTime As TimeSpan
For Each chkBakeItem In gbxBakeryItems.Controls
If chkBakeItem.Checked = True Then
Dim OpStr As String = chkBakeItem.Text ' get the operation string, which will include the time.
' Parse the time -- the number between "( )" as minutes: seconds:
Dim P As Integer = OpStr.IndexOf("("c) ' get the position of the "("
Dim Q As Integer = OpStr.IndexOf(")"c) ' get the position of the "("
' add "00:" at the beginning. This will make a number appear as a minute.
ElapseTime = ElapseTime.Add(TimeSpan.Parse("00:" & OpStr.Substring(P + 1, Q - P - 1)))
' Create a string from "EventTime".
Dim MarkTime As String = Format(EventTime, "hh:mm")
' Index will probably have to be changed, but here it is the next entry.
Dim Index As Integer = lbxOven1.Items.Count
' Insert "hh:mm text" into the listbox.
lbxOven1.Items.Insert(Index, (MarkTime + (" ") & (chkBakeItem.Text)))
' Now add the elapsed time to the "Event Timer". Note that this is done after writing the starting time to the listbox string.
EventTime = EventTime.Add(ElapseTime)
End If
Next
|
|
 |
New Member
|
|
May 9, 2009, 01:09 AM
|
|
Thanks perito,
I'm getting an error message saying: "option strict on disallows late binding" for the line with chkBakeItem.Checked and both lines with chkBakeItem.Text.
Also, I tried it with option strict off and instead of adding just the time of the item before it added all up. e.g.
9:00 Knot Rolls (15)
9:15 Dinner Rolls (18)
9:48 Round Rolls (18) - should be 9:33
I need to have option strict turn on so turning it off isn't an option.
Thanks
|
|
 |
New Member
|
|
May 9, 2009, 01:19 AM
|
|
And yes gbxBakeryItems is a groupbox for the bakery items
|
|
 |
Ultra Member
|
|
May 9, 2009, 04:39 AM
|
|
I'm getting an error message saying: "option strict on disallows late binding" for the line with chkBakeItemChecked and both lines with chkBakeItem.Text.
I'm not sure why it thinks it's using late binding. Are you using Visual Studio 2008 or an earlier version?
Are you creating the checkboxes at run time or are they created at compile time? In my test code, I have Option Strict On, and Option Explicit On. I dropped three checkboxes on the groupbox and put the time in parentheses just like you do, but my debugger doesn't complain.
Also, I tried it with option strict off and instead of adding just the time of the item before it added all up. e.g.
9:00 Knot Rolls (15)
9:15 Dinner Rolls (18)
9:48 Round Rolls (18) - should be 9:33
I found the problem. Change the line that says
ElapseTime = ElapseTime.Add(TimeSpan.Parse("00:" & OpStr.Substring(P + 1, Q - P - 1)))
To
ElapseTime = TimeSpan.Parse("00:" & OpStr.Substring(P + 1, Q - P - 1))
We don't want to add to ElapseTime, we just want ElapseTime to contain the length of time required to bake. The original line was simply adding the bake time to ElapseTime. It might be better to change the name of ElapseTime to BakingTime or OvenTime as it would indicate what we're using it for.
|
|
 |
New Member
|
|
May 9, 2009, 04:43 AM
|
|
I'm using 2008 express edition, and they are created at compile time because on the interface
|
|
 |
Ultra Member
|
|
May 9, 2009, 04:57 AM
|
|
Try using the CType or DirectCast function
CType(chkBakeItem.Checked, Boolean)
Or, if it gags on that, try casting chkBakeItem
CType(chkBakeItem, Checkbox).Checked
|
|
 |
New Member
|
|
May 9, 2009, 05:21 AM
|
|
I worked it out. Turned out I I hadn't declared something earlier in my code.
Still having problems with the time though. Its not adding the times up properly. Instead of adding the time from just the item before its adding all times before. e.g. instead of the times being 9:00, 9:15, 9:30 etc its 9:00, 9:15, 9:45.
And when I added the index in its placing them backwards like:
9:00 Turn on, heat (15)
9:45 Knot Rolls (15)
9:15 Supreme Pizza Rolls (15)
9:00 Hawaiin Pizza Rolls (15)
Ive placed the index number like this:
LbxOven1.Items.Insert(1, (MarkTime + (" ") & (chkBakeItem.Text)))
Down the bottom of the code. Replaced the work "Index" With "1"
Was that right?
|
|
 |
Ultra Member
|
|
May 9, 2009, 05:36 AM
|
|
 Originally Posted by dan28
And when i added the index in its placing them backwards like:
9:00 Turn on, heat (15)
9:45 Knot Rolls (15)
9:15 Supreme Pizza Rolls (15)
10:00 Hawaiian Pizza Rolls (15)
Ive placed the index number like this:
lbxOven1.Items.Insert(1, (MarkTime + (" ") & (chkBakeItem.Text)))
Down the bottom of the code. Replaced the work "Index" With "1"
was that right?
The "1" will place the line in that position in lbxOven1.item list. The first item is "0", the second item is "1", the third item is "2", etc. There's something wrong that I don't understand. The idea of using
Dim Index As Integer = lbxOven1.Items.Count
is that lbxOvem1.Items.Count is always 1 greater than the number of items already in the list. If there are two items in the list, they will have indices of 0 and 1. Count will be 2.
I noticed, myself, when I tried it once that it put them in reverse order. I stopped and restarted and it put them in normal order. That didn't make a lot of sense to me. I looked at it again, and it appears that it's the GroupBox that's somehow reversing the order. The "For Each ..." is sometimes processing them in the wrong order!
I assume that the VS2008 Express (I'm using VS2008 Professional) edition's debugger works the same as my debugger. Walk through the code one line at a time and keep an eye on the variables. Index should proceed 0, 1, 2,. Unfortunately, you have to look at lbxOven1.Items.Item(#) manually. (Highlight "lbxOven1.Item.Items(" and use QuickWatch. Enter the item # and a right parenthesis, "0)" for example. You can then examine exactly where the item entered in the list. Maybe you can figure it out using that.
The alternative is to do what I suggested on the previous thread. Keep track of all of the oven items and their times in a separate array. Then, each time you want to add something, you can use
lbxOven1.Items.Add(... )
and you can build the string inside the parentheses. If you add an item between two other items, or if you subtract an item, you'll have to parse all of the lines from the insertion point to the end. It can be simpler, unless you have a huge list, to use an external array containing the items, and empty and refill the listbox as required.
|
|
 |
New Member
|
|
May 18, 2009, 12:13 AM
|
|
I used Ur code and the time calculate worked with checkbox items,
However, I just wonder can u help me to do calculate items within Listbox?
|
|
Question Tools |
Search this Question |
|
|
Add your answer here.
Check out some similar questions!
Israel, the chosen people of God!Rejected or not?
[ 81 Answers ]
Israel is the chosen people of God. Many Christians believe that Israel is rejected by God because the Jews did not accept Jesus as the Messiah.
Yes , they did not accept Him , but they were the first ones to believe in Him. They believed in His death , they believed in His resurrection.They...
Chosen by the birthmother, BUT.
[ 11 Answers ]
My wife and I have been chosen by a birthmother to adopt her baby girl. One huge obstacle--the birth father. The conception was out of wedlock and in her words, he has not had anything to do with the pregnancy. She states that he is a daily marijuana user and lives a 'hard life' and has always...
The president is chosen by
[ 1 Answers ]
The president is chosen by
a. popular vote.
b. the House of Representatives.
c. state legislatures.
d. electoral college.
View more questions
Search
|