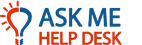 |
|
|
 |
Ultra Member
|
|
Apr 1, 2009, 09:02 AM
|
|
repeating issues
hello
I can't figure out how to get part of my form to repeat
this is what I have at the moment:
Public Class Form5
Dim randomnum As New Random
Dim iNum1 As Integer
Dim iNum2 As Integer
Dim iNum3 As Integer
Dim iNum4 As Integer
Private Sub Form5_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
iNum1 = randomnum.Next(1, 301)
iNum2 = randomnum.Next(1, 301)
iNum3 = randomnum.Next(1, 301)
iNum4 = randomnum.Next(1, 301)
Label2.Text = pupilName
Label4.Text = iNum1
Label6.Text = iNum2
Label8.Text = iNum3
Label10.Text = iNum4
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
'create random numbers all over again for questions 5 times over'
For I = 0 To 5
Me.Form5_Load()
Next
Form6.ShowDialog()
End Sub
Private Sub Question1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Question1.TextChanged
If iNum1 + iNum2 = CInt(Question1.Text) Then
Total = Total + 1
End If
End Sub
Private Sub Question2_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Question2.TextChanged
If iNum3 - iNum4 = CInt(Question2.Text) Then
Total = Total + 1
End If
End Sub
End Class
I am trying to get get the random numbers to regenerate again after the button is clicked, I want it to do this 5 times, before moving onto the next form.
also every time the button is clicked if the answers to the questions are right I want to add 1 to their total score.
any help or hints would be greatly appreciated
thanks :)
|
|
 |
Ultra Member
|
|
Apr 1, 2009, 09:18 AM
|
|
right I've sused out how to get the random numbers to regenerate but now I'm stuck on getting it to only do it 5 times before moving on to the next form:
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
'create random numbers all over again for questions 5 times over'
For I = 0 To 5
iNum1 = randomnum.Next(1, 301)
iNum2 = randomnum.Next(1, 301)
iNum3 = randomnum.Next(1, 301)
iNum4 = randomnum.Next(1, 301)
Label4.Text = iNum1
Label6.Text = iNum2
Label8.Text = iNum3
Label10.Text = iNum4
Next
'Form6.ShowDialog()'
'Me.Close()'
End Sub
|
|
 |
Ultra Member
|
|
Apr 2, 2009, 05:08 AM
|
|
I'm a little unsure of what you're trying to do, but I think what is happening is that you're effectively recreating the form each time you call Form5_Load. You probably need to insert a "Randomize" call somewhere early in your code. This generates a "seed" to make sure the series of random numbers doesn't repeat.
Put Randomize() at the top of your "Loadit" function and see if that doesn't work. If not, post again and I'll help you out.
By the way, what version of Visual Basic are you using?
===========
What are you trying to get to repeat 5 times?
You should be able to keep a class-level variable to keep a count of the number of times it repeats. You can use that variable to prevent the program from closing (FormClosing event, or its equivalent in the version of VB you are using) until the five repeats are accomplished.
|
|
 |
Ultra Member
|
|
Apr 2, 2009, 09:18 AM
|
|
this is now what it looks like (im not sure where you want me to put the randomise?)
Public Class Form5
Dim randomnum As New Random
Dim iNum1 As Integer
Dim iNum2 As Integer
Dim iNum3 As Integer
Dim iNum4 As Integer
Dim I As Integer
Private Sub Form5_Load(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles MyBase.Load
iNum1 = randomnum.Next(1, 301)
iNum2 = randomnum.Next(1, 301)
iNum3 = randomnum.Next(1, 301)
iNum4 = randomnum.Next(1, 301)
Label4.Text = iNum1
Label6.Text = iNum2
Label8.Text = iNum3
Label10.Text = iNum4
End Sub
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
'create random numbers all over again for questions 5 times over'
Question1.Clear()
Question2.Clear()
For I = 1 To 5
If I < 6 Then
iNum1 = randomnum.Next(1, 301)
iNum2 = randomnum.Next(1, 301)
iNum3 = randomnum.Next(1, 301)
iNum4 = randomnum.Next(1, 301)
Label4.Text = iNum1
Label6.Text = iNum2
Label8.Text = iNum3
Label10.Text = iNum4
Else
Form6.ShowDialog()
Me.Close()
End If
Next I
End Sub
Private Sub Question1_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Question1.TextChanged
If iNum1 + iNum2 = CInt(Question1.Text) Then
Total = Total + 1
End If
End Sub
Private Sub Question2_TextChanged(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Question2.TextChanged
If iNum3 - iNum4 = CInt(Question2.Text) Then
Total = Total + 1
End If
End Sub
End Class
I'm using visual studio 05 so I guess its VB 05 then.
I'm trying to get the user to click the button to refresh the numbers(in the labels) so that they can do more sums, I want this to repeat a maximum of 5 times before going onto the next form
I also want to add 1 to the total each time they got a question right, so I can show it at the end(not sure how to do this either but first things irst)
|
|
 |
Ultra Member
|
|
Apr 2, 2009, 06:53 PM
|
|
Put the Randomize statement in the Form5_Load subroutine. Take everything else out of that subroutine and put it in a separate subroutine like this:
Private Sub GenerateRandom()
iNum1 = randomnum.Next(1, 301)
iNum2 = randomnum.Next(1, 301)
iNum3 = randomnum.Next(1, 301)
iNum4 = randomnum.Next(1, 301)
Label2.Text = pupilName
Label4.Text = iNum1
Label6.Text = iNum2
Label8.Text = iNum3
Label10.Text = iNum4
End Sub
Declare a class-level variable, Counter
Dim Counter as integer
In Form5_Load, call this subroutine. Form5_Load will look like this:
Private Sub Form5_Load
Randomize
Counter = 0 ' this will zero the counter each time the form is loaded.
GenerateRandom
End Sub
In Button1_Click, call GenerateRandom
Private Sub Button1_Click
if Counter < 5 then
Question1.Clear()
Question2.Clear()
GenerateRandom
Counter = Counter + 1 ' Count the number of times that he clicks here.
Else
Form6.ShowDialog
Close
' Do what you want to do after the counter = 5
End If
End Sub
Each time the button is pressed, Button1_Click is called. That will clear the questions and Generate the random values. It's a little more professional to put that in a separate subroutine and call that from various places. Calling Form5_Load several times isn't "good form". You should only put things in the "Form Load" subroutine that are needed when the form actually loads.
It might be better to call "Form6.ShowDialog" from the same place that Form5 is called, rather than calling it from Form5. If Form5 is called as a "modal" window (ShowDialog) you'll have something like this: (the right-side of the comparison isn't correct syntax, but I don't have VB loaded and I can't remember what the Dialog Result constants are. This is more VB.NET-like. Hopefully, you'll understand. If you really need the constants, I can look them up for you.)
If Form5.ShowDialog = DialogResult.OK then
Form6.ShowDialog
End If
|
|
Question Tools |
Search this Question |
|
|
Check out some similar questions!
Count in excel excluding repeating numbers
[ 1 Answers ]
Is there a way for me to count in a column and exclude numbers that repeat? I am working a spreadsheet with account numbers and need to count how many account numbers... the problem is that some of them repeat within the spreadsheet. Can someone help?
Thanks in advance!
Repeating the same mistake
[ 4 Answers ]
I have a problem which has I've repeated all my life.
I just split from a girl after 4 years. The relationship was very volatile, passionate, but ultimately , unhealthy and going nowhere. But we both love each other.
After ending it, and making that descison, I have massive regret, now wanting...
Repeating
[ 3 Answers ]
How many different ways can 8 letters be grouped with no letter being repeated?
A Repeating Application Error Message.
[ 2 Answers ]
FONT-Courier NewSIZE=3COLOR=SlateGrey :confused:
SUBJECT: A Repeating application error message.
1. Description of the problem:
Several times a day while I'm working at my computer,
the following occurs. I click on an icon
or try the use Mozilla FireFox v1.0.2.
The...
View more questions
Search
|