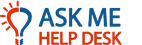 |
|
|
 |
New Member
|
|
Feb 18, 2009, 06:22 AM
|
|
Calculating Earnings per Share
As an assignment we have to calculate the earnings per share and earnings yield. I am using Visual C++ to create this calculator. The calculator has to be in a form with the correct buttons. I created the form but after writing the code I keep getting NaN as the answer. This is what I've written:
// Code for calculating Earnings per Share
double earnings;
double profit;
double shares;
earnings = Convert::ToDouble(tbProfit->Text);
earnings = profit;
earnings = Convert::ToDouble(tbShares->Text);
earnings = profit / shares;
tbEarnings->Text = Convert::ToString(earnings);
tbEarnings->Text = earnings.ToString(".00");
//Code for Calculating Earnings Yield
double price;
double yield;
yield = Convert::ToDouble(tbEarnings->Text);
yield = earnings;
yield = Convert::ToDouble(tbPrice->Text) / price;
yield = (earnings / price) * 100;
tbYield->Text = Convert::ToString(yield);
tbYield->Text = yield.ToString(".00");
After it compiles, the Earnings per Share and Earnings Yield text box show "NaN". Am not sure where I went wrong. I would appreciated any help given. This assignment is due in a couple hours and I don't what to do anymore.
Thank you
|
|
 |
Full Member
|
|
Feb 18, 2009, 07:07 AM
|
|
You're not assigning values to your variables correctly.
earnings = Convert::ToDouble(tbProfit->Text);
earnings now contains the Profit value found in the text box.
earnings = profit;
earnings now contains a zero.
earnings = Convert::ToDouble(tbShares->Text);
earnings now contains the Shares value found in the text box.
earnings = profit / shares;
profit is a zero and shares is a zero. Nothing has been assigned to either. This will give a NaN result.
------------------------
Try this:
double earnings;
double profit;
double shares;
profit = Convert::ToDouble(tbProfit->Text);
shares = Convert::ToDouble(tbShares->Text);
earnings = profit/shares; (you need to protect this by putting an IF statement around it to make sure that shares > 0).
tbEarnings->Text = earnings.ToString(".00");
double price;
double yield;
earnings = Convert::ToDouble(tbEarnings->Text);
price = Convert::ToDouble(tbPrice->Text)
yield = (earnings / price) * 100; (You should also protect with an IF statement to make sure that price > 0)
tbYield->Text = yield.ToString(".00");
|
|
 |
New Member
|
|
Feb 18, 2009, 07:38 AM
|
|
Thank you!! Thank you!!
It worked! I'm really new at this thing. I did last semester but I'm very disappointed because the professor never really taught the subject well. Now am stuck again with it and once again am clueless. But thanks very much.
Now I have to do a couple "If" statements if the user does not input certain values. If Profit and Shares are missing but EPS is provided then calculate EY. If either Profit or Shares is missing and EPS is missing then an error message is displayed. If Price is missing display error message.
Let's see how this goes.
Hope you're still around if I get stuck again :)
Thanks again
|
|
 |
New Member
|
|
Feb 18, 2009, 08:19 AM
|
|
Ok I'm stuck again. I have no idea how to do the If statement - If either Profit or Shares or both are missing but EPS is provided then use EPS to calculate EY.
|
|
 |
Full Member
|
|
Feb 18, 2009, 09:49 AM
|
|
if (shares > 0) {
earnings = profit/shares;
}
|
|
 |
New Member
|
|
Feb 18, 2009, 10:05 AM
|
|
Thanks. I tried that but it didn't work
|
|
 |
Full Member
|
|
Feb 18, 2009, 10:07 AM
|
|
It is the correct syntax. It has to work.
double earnings;
double profit;
double shares;
profit = Convert::ToDouble(tbProfit->Text);
shares = Convert::ToDouble(tbShares->Text);
if (shares > 0) {
earnings = profit/shares;
}
tbEarnings->Text = earnings.ToString(".00");
double price;
double yield;
earnings = Convert::ToDouble(tbEarnings->Text);
price = Convert::ToDouble(tbPrice->Text)
if (price != 0) {
yield = (earnings / price) * 100;
}
tbYield->Text = yield.ToString(".00");
Note that on the second set, I put a "!=" (not equal). That can also be used.
|
|
 |
New Member
|
|
Feb 18, 2009, 10:18 AM
|
|
Ok I tried that but what I want to happen is if the user does not enter a value for profit or shares but there is a value for EPS then you should still be able able to calculate the Yield
|
|
 |
Full Member
|
|
Feb 18, 2009, 10:29 AM
|
|
I don't see an EPS value in the code so far, but if I understood you correctly, you can always add some code for an "else" box:
if () {
this is the code that will be executed if the if statement is true.
}
else
{
this is the code that will be executed if the if statement is false
}
This is what we call "defensive programming". If the user does not enter a value for profit or shares, you can't calculate earnings or yield. The code so far will put a "0" in for those values.
I know that in C# and other .NET languages, yield and earnings will have default values of 0. I'm not positive about it in C or C++ so you may want to try it to see if it's still true. Back in the MS-DOS days, it would not have been true. Whatever value was on the "stack" (where temporary variables are created) would be the value printed.
|
|
 |
New Member
|
|
Feb 18, 2009, 10:35 AM
|
|
I'm sorry if I missled you but EPS is actually earnings. If the user leaves the profit or shares or both empty yet they provide and input for earnings, then the program should calculate the yield.
|
|
 |
Full Member
|
|
Feb 18, 2009, 10:41 AM
|
|
Well, you'll have to provide the formula to calculate earnings if shares is 0. The code will be something like this
if (shares > 0) {
earnings = profit/shares;
}
else {
earnings = 10.00 // or some other calculation.
}
tbEarnings->Text = earnings.ToString(".00");
|
|
 |
New Member
|
|
Feb 18, 2009, 10:53 AM
|
|
That's the problem. You need shares to calculate earnings. I don't know. :( I'm frustrated now.
|
|
 |
New Member
|
|
Feb 18, 2009, 11:44 AM
|
|
Thanks a lot for your help though. I'm just going to hand it what I did. I'm at a lost right now. I don't know what else to do
|
|
Question Tools |
Search this Question |
|
|
Add your answer here.
Check out some similar questions!
Calculating net earnings and preparing statement of retained earnings
[ 2 Answers ]
I just started my introduction to accounting class in university and I am a little stuck on this question because we didn't really go over this is class... please help!
Here is the information given: Sea Surf Campground is a public camping ground in Ocean National Park. It has the following...
Calculating diluted earnings per share
[ 0 Answers ]
How is diluted earnings per share calculated? Is all preferred stock convertible to common or only if it is called convertible preferred? I am trying to answer a question in my hw. It lists preferred stock (doesnt say it is convertible), common stock and stock options. I know I need to account...
View more questions
Search
|